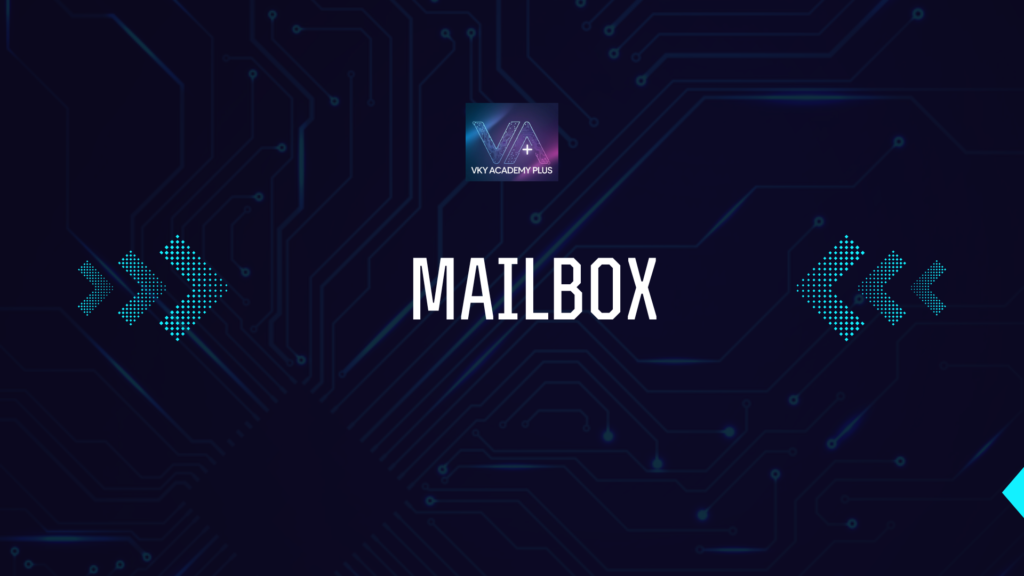
Mailbox in SystemVerilog
A mailbox in SystemVerilog is a communication mechanism used for passing data between different processes in a testbench environment. It allows processes to send and receive data asynchronously, making it a powerful tool for inter-thread communication.
Why is Mailbox Used?
- Mailboxes enable efficient synchronization between producer and consumer processes.
- They provide buffered storage for data, allowing flexibility in transaction-based testbenches.
- They help implement a message-passing mechanism for components that do not share direct variables.
What Technique Does Mailbox Use?
- The mailbox operates using a FIFO (First In, First Out) queue structure, ensuring that messages are retrieved in the same order they were sent.
- It supports both blocking and non-blocking operations:
- Blocking: The receiver waits until data is available.
- Non-blocking: The receiver checks if data is available but does not wait.
Mailbox Methods in SystemVerilog
A mailbox in SystemVerilog is a built-in class used for communication between processes, following a FIFO (First In, First Out) mechanism. It provides various methods to send and receive messages efficiently.
Important Mailbox Methods:
- new()
- Creates a new mailbox.
- Example:
mailbox my_mailbox = new();
- put()
- Places an item into the mailbox.
- Blocking if the mailbox has a size limit and is full.
- Example:
my_mailbox.put(data);
- get()
- Retrieves an item from the mailbox.
- Blocking if the mailbox is empty (waits until data is available).
- Example:
my_mailbox.get(received_data);
- try_put()
- Attempts to place an item in the mailbox without blocking.
- Returns 1 if successful, 0 if the mailbox is full.
- Example:
if(my_mailbox.try_put(data)) $display("Data sent!");
- try_get()
- Attempts to retrieve an item without blocking.
- Returns 1 if successful, 0 if the mailbox is empty.
- Example:
if(my_mailbox.try_get(received_data)) $display("Data received!");
- peek()
- Similar to
get()
, but does not remove the item from the mailbox. - Example:
my_mailbox.peek(peeked_data);
- Similar to
- num()
- Returns the number of items currently in the mailbox.
- Example:
int count = my_mailbox.num();
- size()
- Returns the maximum capacity of the mailbox.
- Example:
int max_size = my_mailbox.size();
Real-Life Analogy for Mailbox
Imagine a real-world post office mailbox:
- People drop letters (data) into the mailbox.
- The postman (receiver) collects the letters in the order they were dropped (FIFO order).
- If the mailbox is empty, the postman can either wait (blocking) or check later (non-blocking).
Mailbox Example:
class pkt;
rand bit [4:0] addr;
endclass
typedef enum {pass, fail} state_t;
mailbox #(string) smbox = new; //creates a new mailbox named smbox that can hold items of type string
mailbox #(pkt) pmbox = new; //creates a new mailbox named pmbox that can hold items of type pkt
mailbox #(state_t) tmbox = new; //creates a new mailbox named tmbox that can hold items of type state_t
module tb;
initial begin
pkt ppkt = new;
string pstring;
state_t pstatus;
pstring = "test one";
assert(ppkt.randomize());
pstatus = pass; // Assign a value to pstatus
smbox.put(pstring);
pmbox.put(ppkt);
tmbox.put(pstatus);
$display("====================== PUT THE DATA ================================");
$display("Put into smbox: %s", pstring);
$display("Put into pmbox: addr=%0d", ppkt.addr);
$display("Put into tmbox: %s", pstatus.name());
end
initial begin
string gstring;
pkt gpkt;
state_t gstatus;
smbox.get(gstring);
pmbox.get(gpkt);
tmbox.get(gstatus);
$display("====================== GET THE DATA ================================");
$display("Got from smbox: %s", gstring);
$display("Got from pmbox: addr=%0d", gpkt.addr);
$display("Got from tmbox: %s", gstatus.name());
end
endmodule
Output:
====================== PUT THE DATA ================================
Put into smbox: test one
Put into pmbox: addr=16
Put into tmbox: pass
====================== GET THE DATA ================================
Got from smbox: test one
Got from pmbox: addr=16
Got from tmbox: pass
The output shows that:
- The string “test one” was successfully stored and retrieved.
- The randomly generated address 16 was stored and retrieved correctly.
- The enumeration value pass was stored and retrieved as expected.
Understanding the code:
This SystemVerilog testbench demonstrates how mailboxes are used for communication between different processes. The mailbox acts as a storage unit, allowing one process to send data while another retrieves it asynchronously. This approach is commonly used in verification environments to handle inter-process communication.
Class Definition (pkt
)
The code defines a class named pkt
, which contains a randomizable 5-bit address field. The rand
keyword allows the field to be assigned a random value whenever randomize()
is called. This is useful for generating test scenarios where addresses need to vary dynamically.
Enumeration (state_t
)
An enum type state_t
is defined with two possible values: pass
and fail
. This represents different status conditions, which can be stored and retrieved from the mailbox.
Mailboxes Declaration
Three different mailboxes are declared to store different types of data:
smbox
stores strings.pmbox
stores objects of thepkt
class.tmbox
storesstate_t
enumeration values.
Mailboxes facilitate communication between different parts of the testbench by storing data that can be retrieved later.
First initial
Block – Writing to Mailboxes
In this block:
- A new instance of the
pkt
class is created. - A string variable is assigned the value
"test one"
. - The
randomize()
function assigns a random 5-bit address to thepkt
object. - The
state_t
variable is assigned the valuepass
. - The put() method stores the string, class object, and enumeration value into their respective mailboxes.
- A display message confirms that the values have been successfully stored.
Second initial
Block – Reading from Mailboxes
This block retrieves the stored values using the get() method:
- A string variable retrieves the stored message.
- A
pkt
object retrieves the previously stored randomized address. - A
state_t
variable retrieves the stored enumeration value. - A display message confirms that the correct values have been retrieved.
FIFO Behavior of Mailboxes
Mailboxes in SystemVerilog follow the First-In, First-Out (FIFO) principle. This means that:
- The first value placed into a mailbox is the first one retrieved.
- The order of retrieval matches the order in which values were stored.
Real-Life Analogy
The mailbox system in this code works similarly to a postal system:
- One person drops a letter (put) into a mailbox.
- Later, another person retrieves the letter (get).
- The mailbox ensures that letters are retrieved in the same order they were dropped in.
Let us understand the concept of mailbox with the help of another code:
Code Example-2
Imagine a small town post office where letters and parcels arrive daily, waiting to be delivered to the right recipients. Each day, the postmaster carefully sorts them into separate mailboxes—one for letters and another for parcels. Our SystemVerilog code mimics this real-world process using mailboxes to handle different types of messages efficiently.
In our setup, there are different kinds of mail. Letters come in various forms, such as postcards and telegrams, each carrying different types of messages. Similarly, parcels come in other categories, like food parcels filled with fresh fruits or anything else and toy parcels carrying delightful surprises. These various types of letters and parcels are modeled as classes, with letters and parcels serving as the base categories and postcards, telegrams, food parcels, and toy parcels as specialized versions.
Once the mail items are ready, the postmaster places them into their respective mailboxes. The letter mailbox holds all the letters, whether they are postcards or telegrams, while the parcel mailbox stores food and toy parcels. Each time a mail item is placed in a mailbox, a message is printed to confirm that it has been successfully stored. This ensures that nothing gets lost in transit.
But what happens next? Just like a mail carrier who collects letters and parcels for delivery, our code retrieves the stored messages one by one. When an item is taken from a mailbox, the system announces what was received, ensuring that every letter and parcel reaches its destination without mix-ups.
The result of this simulation mirrors what happens in real life. First, we see messages confirming that the mail has been placed into the mailbox. Later, we observe the retrieval process, where the mail is collected and read in the same order it was stored. This demonstrates the FIFO (First In, First Out) nature of mailboxes—just like how letters and parcels are picked up in the order they arrived.
By structuring the code in this way, we achieve an efficient and organized way to handle communication between different parts of a testbench in SystemVerilog, making sure that data flows smoothly, just like a well-run post office!
class Letter;
string content;
function new(string content = "");
this.content = content;
endfunction
endclass
class Postcard extends Letter;
function new(string content = "");
super.new(content);
endfunction
endclass
class Telegram extends Letter;
function new(string content = "");
super.new(content);
endfunction
endclass
class Parcel;
string description;
function new(string description = "");
this.description = description;
endfunction
endclass
class FoodParcel extends Parcel;
function new(string description = "");
super.new(description);
endfunction
endclass
class ToyParcel extends Parcel;
function new(string description = "");
super.new(description);
endfunction
endclass
mailbox #(Letter) letter_mbox = new;
mailbox #(Parcel) parcel_mbox = new;
module mailbox_example;
initial begin
Postcard postcard = new("Greetings from India!");
Telegram telegram = new("Urgent: Meeting at 3 PM");
FoodParcel food_parcel = new("Fresh fruits and vegetables");
ToyParcel toy_parcel = new("Toy cars and Bikes");
$display("=============Putting the Item in the Mailbox ===========");
letter_mbox.put(postcard);
$display("Putting letter into mailbox: %s", postcard.content);
letter_mbox.put(telegram);
$display("Putting letter into mailbox: %s", telegram.content);
parcel_mbox.put(food_parcel);
$display("Putting parcel into mailbox: %s", food_parcel.description);
parcel_mbox.put(toy_parcel);
$display("Putting parcel into mailbox: %s", toy_parcel.description);
end
initial begin
Letter received_letter;
Parcel received_parcel;
$display("============= Getting the Item from the Mailbox ===========");
letter_mbox.get(received_letter);
$display("Received letter: %s", received_letter.content);
letter_mbox.get(received_letter);
$display("Received letter: %s", received_letter.content);
parcel_mbox.get(received_parcel);
$display("Received parcel: %s", received_parcel.description);
parcel_mbox.get(received_parcel);
$display("Received parcel: %s", received_parcel.description);
end
endmodule
Output:
=============Putting the Item in the Mailbox ===========
Putting letter into mailbox: Greetings from India!
Putting letter into mailbox: Urgent: Meeting at 3 PM
Putting parcel into mailbox: Fresh fruits and vegetables
Putting parcel into mailbox: Toy cars and Bikes
============= Getting the Item from the Mailbox ===========
Received letter: Greetings from India!
Received letter: Urgent: Meeting at 3 PM
Received parcel: Fresh fruits and vegetables
Received parcel: Toy cars and Bikes
Understanding the Output Step by Step:
1. Putting the Items in the Mailbox
The first section of the output confirms that four different items—a combination of letters and parcels—are successfully placed in their respective mailboxes. Each time an item is stored, a message is printed to indicate its placement:
- A postcard with the message “Greetings from India!” is placed into the letter mailbox.
- A telegram with the message “Urgent: Meeting at 3 PM” follows, also being placed in the letter mailbox.
- A food parcel containing “Fresh fruits and vegetables” is added to the parcel mailbox.
- Lastly, a toy parcel containing “Toy cars and Bikes” is stored in the parcel mailbox.
This ensures that the mailboxes are populated in the correct order and are ready for retrieval.
2. Getting the Items from the Mailbox
The second section of the output confirms that the stored items are retrieved in the same order they were placed. Since mailboxes operate in FIFO (First In, First Out) order, the items are collected in sequence:
- The first letter retrieved is the postcard, displaying “Greetings from India!”
- The second letter retrieved is the telegram, displaying “Urgent: Meeting at 3 PM”
- The first parcel retrieved is the food parcel, displaying “Fresh fruits and vegetables”
- The final parcel retrieved is the toy parcel, displaying “Toy cars and Bikes”
This output verifies that data integrity is maintained throughout the process. Each item is successfully placed into the mailbox, stored temporarily, and retrieved without errors. The messages confirm that the FIFO principle is followed—ensuring an orderly process, just like how real-world post offices handle mail sorting and delivery.
Understanding Mailbox in a SystemVerilog Testbench Environment
In a SystemVerilog-based dynamic testbench environment, a mailbox serves as a communication channel between different testbench components. It allows stimulus data, transactions, and control signals to be passed efficiently between modules, enabling synchronization between the testbench and the Device Under Verification (DUV).
What Exactly Are We “Mailing” in a System Verilog Testbench?
Think of a mailbox as a post office where different types of mail (data packets, commands, responses) are exchanged between sender and receiver entities. In a SystemVerilog testbench, the mailbox is used for transferring transactions, control signals, or stimulus data between different components, such as:
- Testbench to Driver (Transmitting generated stimulus)
- Driver to Monitor (Observing responses from the DUV)
- Monitor to Scoreboard (Comparing expected and actual responses)
- Between BFMs (Bus Functional Models) (For protocol handling)
Each transaction, which represents a meaningful data exchange, is treated like a “letter” or “parcel” that gets stored in the mailbox until it is retrieved by the appropriate component.
Explanation of Testbench Architecture :
The testbench consists of several critical components that interact dynamically:
- Tests and Generator
- The Tests define different scenarios to verify the functionality of the DUV.
- The Generator creates input transactions and feeds them into the testbench.
- Mailboxes for Transaction Handling
- The testbench uses mailboxes to pass generated transactions between components.
- The Coverage Model monitors transaction flow and ensures functional coverage.
- Scoreboard (Reference Model & Comparison Logic)
- A reference model generates expected results.
- Comparison logic checks if the DUV output matches the expected response.
- Write and Read Interfaces
- Write BFM (Bus Functional Model): Sends transactions to the DUV.
- Read BFM: Captures responses from the DUV.
- Monitors observe data flow and send it to the scoreboard for analysis.